
Continuous Integration (CI) and Continuous Delivery (CD) is the most efficient approach to integrating the work of different team members into a single performing product. Setting up CI/CD helps development teams streamline the entire software development lifecycle (SDLC), from development and testing to delivery and deployment. It integrates the coding efforts of individual developers into a central repository, allowing the changes to merge quickly and easily. It also ensures that all changes made in the production stage are released to the customer. Let us understand some fundamental concepts before getting into further details of building a CI/CD pipeline with Jenkins.
CI or Continuous Integration automates the integration of code changes from multiple developers into a single codebase and frequently committing their work to the central code repository (GitHub or Stash). It helps identify and resolve bugs quicker, ensures that integrating code across a team of developers is easy, and improves software quality at a reduced time. Some popular CI tools are Jenkins, TeamCity, and Bamboo. CD or Continuous Delivery is carried out after Continuous Integration that helps ensure that all new changes are released to customers without errors. This also includes running integration and regression tests in the staging area (like in the production environment); thus, the final release is intact and is not broken in production. Popular CD tools are AWS Code Deploy, Jenkins, and GitLab.CI/CD also ensures that every change is validated via test automation. This helps reduce turnaround times for the feedback and prevents failed tests from being deployed to production.
How does the CI/CD pipeline work, and Why Jenkins?
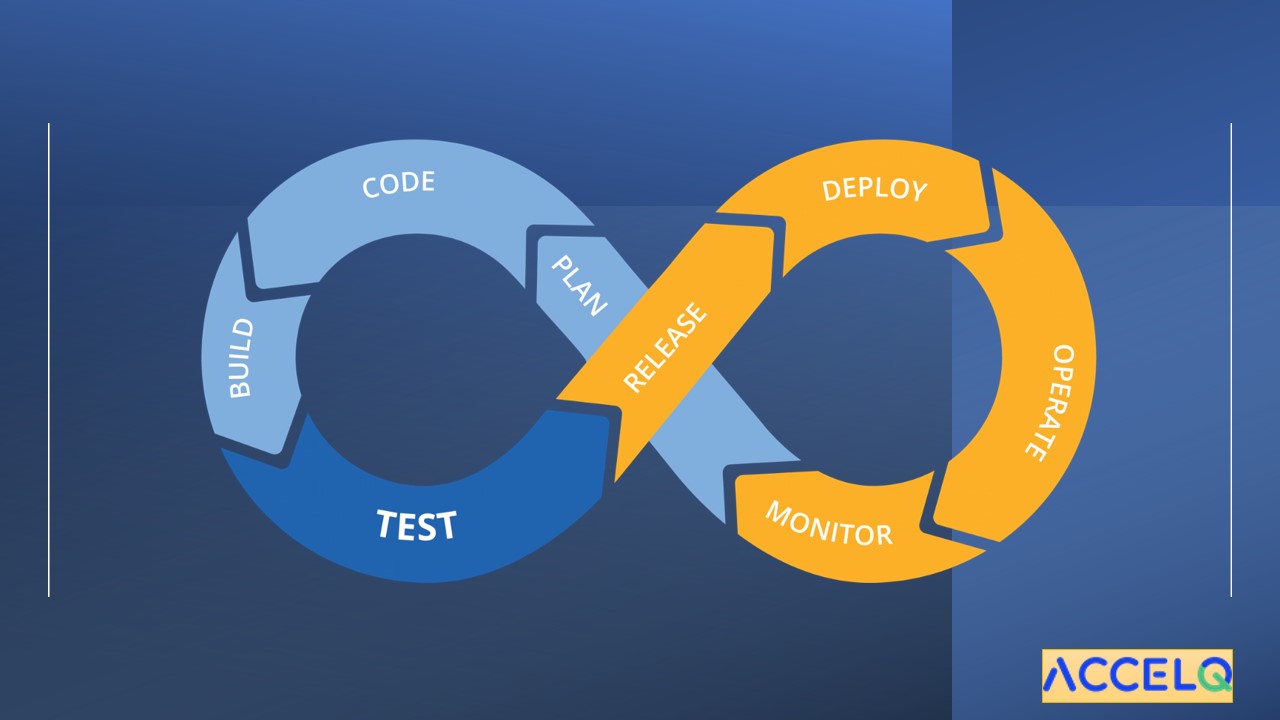
CI/CD is an end-to-end automated process involving steps of frequent code integration, automated testing, and continuous deployment of software changes to production.
1. Code integration; where developers commit their code changes to a remote repository (such as GitHub, GitLab, or BitBucket) and integrated with the main codebase. This helps ensure the code changes align with the rest of the codebase and keep up with the build.
2. Automated testing involves running a suite of tests to check if code changes are functional, pass through expected quality standards, and are free of defects. This can help identify issues early and get developers to fix them quickly and efficiently.
3. Continuous deployment: code changes are automatically deployed to a staging environment to test further. This ensures that software is continuously updated with changes, delivering new features and functionality quickly and efficiently.
4. Production deployment: software changes are released to end-users finally; the production environment is monitored, ensuring that the software functions smoothly, identifying and issues fixed.
These four steps of a CI/CD pipeline work in tandem to ensure that software changes are tested, integrated, and deployed to production seamlessly, helping development teams achieve faster release cycles, reduce risks of software defects, and improve user experience.
SUGGESTED READ - What Is Jenkins in DevOps
Why use Jenkins for CI/CD?
Jenkins is an open-source automation server that facilitates the automation of several stages of the software development lifecycle, such as application development, testing, and deployment.
- It is a server-based application and requires a servlet container like Apache Tomcat to run on various platforms like Windows, Linux, macOS, Unix, etc.
- To use Jenkins, pipelines or a series of steps must be set up for the Jenkins server.
- The Jenkins CI Pipeline is a robust machine consisting of tools designed to host, monitor, and compile test codes /changes.
- It uses a Continuous Integration Server (Jenkins, Bamboo, CruiseControl, TeamCity), a source control tool such as CVS, SVN, GIT, Mercurial, Perforce, ClearCase, a build tool such as Make, ANT, Maven, Ivy, Gradle, and finally an automation testing framework such as Selenium, Appium, TestComplete, UFT, etc.
Getting started with Jenkins:
Jenkins is distributed as a WAR (web archive) file or as installer packages for the major operating systems: Homebrew package, Docker image, and source code. Jenkins is primarily Java-based and supports installation and scaling on Kubernetes and can be executed on the Jenkins WAR standalone or as a servlet in a Java application server such as Tomcat. In any way, it produces a web user interface and accepts calls to its REST API (Application Programming Interface). When running for the first time, Jenkins creates an administrative user with a long random password that can be pasted onto the initial web page to unlock the installation.
1.Download and install Jenkins and choose the platform to run it: Windows, Linux, macOS, or within a Docker container. Initial Setup: The password can be found in the Jenkins console log or in a file specified in the log (typically a secrets/initial AdminPassword file).
2. To Configure Jenkins, access it through a web browser at http://localhost:8080 (or the appropriate URL if not run locally). To install Plugins: During setup, suggested plugins or specific ones can be chosen and installed. For a CI/CD pipeline, plugins such as Git, Pipeline, and Docker can be used, and an admin user for Jenkins can be created to manage access.
3. To create a Job/Project, click "New Item" on the Jenkins dashboard and select project type. Choose 'Freestyle project' for a simple scenario or 'Pipeline' for more complex workflows. Under the Source Code Management tab, select Git (or another version control system) and provide the repository URL and credentials if required.
4. To automate Builds: Under the "Build Triggers" tab, triggering builds can be chosen on SCM (Source Control Management) change (like a Git push) or at regular intervals (using cron syntax).
5. To define the build steps, first add steps that Jenkins should execute, like compiling code, running tests, etc. This will depend on the specific project's language and framework.
6. To set up testing and reporting, automate steps in the build phase and include commands to run tests. For instance, for a Java project, this might be running mvn test. Configure Jenkins to publish test reports. Many testing frameworks are supported with appropriate plugins.
7. To implement Continuous Deployment/Delivery: first is the deployment step; in the Post-build Actions, add steps for deployment. This could be deployed to a staging server or production server or triggering a Docker image build and push to a registry. Pipeline as Code (Optional): For more complex pipelines, use Jenkinsfile to define pipeline as code. This file is checked into the SCM.
8. To configure Notifications: Set up notifications (like email, Slack, etc.) for build success or failure.
9. Regularly check the build success/failure rates and optimize as needed to maintain and monitor builds. Keep Jenkins and its plugins updated for new features and security patches.
10. Ensure Jenkins is secured through proper authentication and authorization settings and regularly back up the Jenkins configuration and data.
The Jenkinsfile, which defines the pipeline as code, is written in Groovy and outlines the stages of the pipeline, such as building, testing, and deploying a given application. Here is a basic example of this file for a Java-based project. This is given based on Git as the version control system and Maven for building the project. The script can be adjusted based on the project's language, tool, and deployment needs.
pipeline {
agent any
environment {
// Define environment variables if needed
MAVEN_HOME = '/path/to/maven'
}
stages {
stage('Checkout') {
steps {
// Checking out the code from the Git repository
checkout scm
}
}
stage('Build') {
steps {
// Building the project with Maven
sh "${MAVEN_HOME}/bin/mvn clean package"
}
}
stage('Test') {
steps {
// Running tests using Maven
sh "${MAVEN_HOME}/bin/mvn test"
}
post {
// Handling test results and artifacts
always {
junit '**/target/surefire-reports/TEST-*.xml'
archiveArtifacts artifacts: '**/target/*.jar', fingerprint: true
}
}
}
stage('Deploy') {
steps {
// Add deployment steps here
// For example, deploying to a staging or production environment
echo 'Deploying application...'
// sh 'deployment-script.sh'
}
}
}
post {
always {
// Actions to perform after the pipeline execution
echo 'Pipeline execution is complete.'
}
}
}
- Pipeline Block: Defines the pipeline and contains all stages.
- Agent: Specifies where the pipeline will run. any means it can run on any available agent.
- Environment: Used to define environment variables.
- Stages: The core part of the Jenkins pipeline. Each stage block can have several steps.
- Checkout: Checks out the source code from the configured source control management (SCM), like Git.
- Build: Compiles the code. Here, it is using Maven (mvn clean package).
- Test: Runs tests and reports the results. This example assumes JUnit tests with Maven.
- Deploy: Deploys the application. This is a placeholder and should be replaced with actual deployment steps, which vary depending on where and how the application is deployed.
- Post: Defines actions that occur after the pipeline stages complete, such as cleanup, notifications, etc.
Remember, this is just a basic example to get started. Setting up a CI/CD pipeline with Jenkins is a great way to get your hands on a quicker and more robust way to integrate your entire chain of build, test, and deployment tools.
Geosley Andrades
Director, Product Evangelist at ACCELQ
Geosley is a Test Automation Evangelist and Community builder at ACCELQ. Being passionate about continuous learning, Geosley helps ACCELQ with innovative solutions to transform test automation to be simpler, more reliable, and sustainable for the real world.
Related Posts
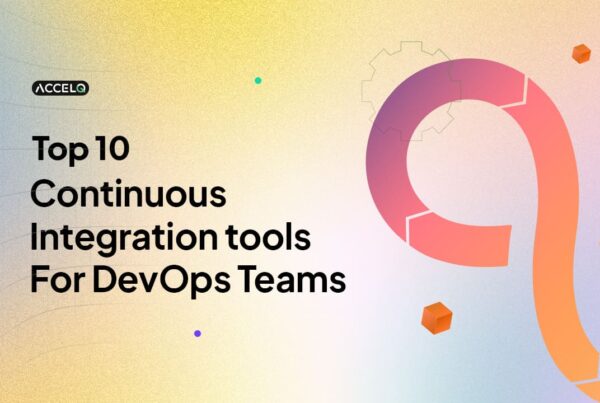
Top 10 Continuous Integration Tools In 2024
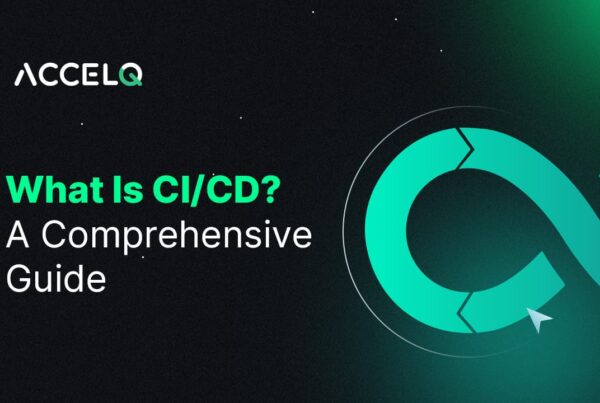